Deck of Cards
This is an implementation of a deck of cards using the OOP paradigm. The purpose of this implementation is to showcase some of the features of object-oriented programming that make it great. The other purpose is to provide a snapshot of my familiarity with OOP and Python at the beginning of this module.
Card Class
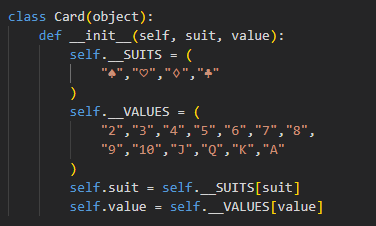
TheCard
class above has two private attributes and two public attributes.
Private attributes are attributes not intended for access from outside an instance.
Public attributes are attributes that are free to be accessed from ouside an instance.
Deck Class

TheDeck
class above inherits from the list class.
Inheritence is a feature of OOP, which allows the adoption of attributes and
methods from one class to another. By inheriting from the list class, instances
of the Deck
class may be passed as an argument to the len()
function,
or followed by the .sort()
list method; for example.
IntegerCard Class
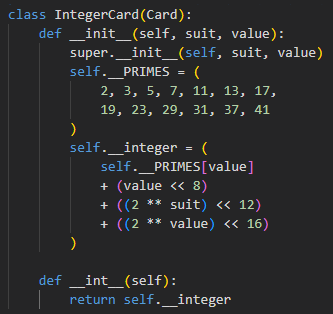
TheIntegerCard
class above inherits from the Card
class
and further abstracts the features of a physical card.
Abstraction is the manipulation of the details of a concept to arrive at
a more general concept, and is a general technique of OOP. Usually abstraction
refers to the creation of abstract classes, which are classes that provide an
interface for other classes to implement.
But in the above example, the IntegerCard
is not an abstract class.
It's an abstraction in the sense that the details of a physical card have been
encoded to a non-human readable format in preparation for an implementation of a
quick hand-ranking algorithm.
PokerHand Class
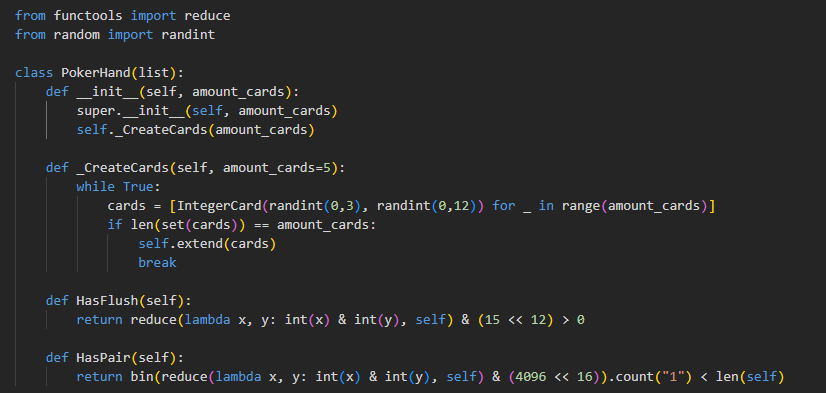
ThePokerHand
class above encapsulates details connected to a physical poker hand,
although it is not yet complete.
Encapsulation is the provision of a clear boundary to something. The objects of
OOP naturally encapsulate information in the form of attributes and methods. A good name for a
class clarifies
it's purpose, for example it's clear that the PokerHand
class represents a
physical poker hand. That doesn't mean classes need to mimic physical objects though. It's also
common
to name classes after design patterns, which are abstractions of structure that is unique
to OOP.
The last thing I'd like to point out is that it's usually good practise to maintain common interfaces for your classes where possible. This can simplify programmes to a large degree, by allowing a single procedure to be enough for a bunch of different objects, which share the same interface. This idea is known as polymorphism.
If you would like to learn more about implementing a game of five card draw poker using OOP, check out my old home project. If you would like to learn more about OOP, then checkout the other module artefacts.
End of Module Reflection
At the start of this module, I had some familiarity with Python and OOP already. The extent of ability was marked by this section, and the linked poker-engine project I'd worked on previously. But I had no previous explicit experience with design patterns or modelling languages.
Now that I'm at the end of this module, I wish I could go back to my old poker project and upgrade it's overall design. During this module, I've learnt some design patterns and python tricks that would be actually useful in that project. For example, In my old implementation, I never thought to encapsulate player actions; but if I had used the command design pattern, it would have simplified the action tracking algorithm that I frankensteined together, and allowed undoing actions when handling errors. During the course, I learnt some Python tricks too, such as using the classmethod decorator to constrain or diversify object initialisations, which could be used to limit a deck to 52 unique cards in theory. I've gained an appreciation of modelling languages too, which helped me reflect on my old poker project, and simplify it's design.
I recognise the benefits of learning object-oriented programming, are not just for my poker project, but extend in to the professional world of software engineering, where I currently work as part of a team. On some level, OOP, design patterns and modelling languages communicative tools for developing ideas as part of research, and on another level they are a set of tried and tested standards underpinning many successful projects. Their popularity and effectiveness makes these topics vital to any software engineer expecting to work with others.
The next step for me professionally I think is to learn another language, such as c++, which is a valuable tool in the software engineering for its speed. That being said, c# might be a good canditate too. Both langauges are connected to game engines, that I could learn as a hobby alongside my current job. I currently work as a game developer, mainly using typescript, so those languages would compliment my cv. I'm especially interested in making games that incorporate ai models, to gain some experience in that field too.